JS Exercise 4: Functions
(Week 9, Tuesday 10/23)
In computer programming, a function is a named section of a program that perform a specific task; they are basically “mini programs” within a larger program. Those functions can then be called at any point, rather than simply running when the page initially loads. In this exercise, we are going to learn about how to write functions in JavaScript.
Start by downloading and uncompressing the function-examples.zip folder. We will be referring to example pages in that folder throughout this exercise.
Function Declarations
Open functions1.html in your editor and look at the code. It contains several examples of JavaScript function declarations. Note that when we declare a JavaScript function, we do not declare the type of the arguments it will accept, nor the type of the value it returns.
Preview the file it in a browser and check the console output; make sure you understand what's happening.
Function Expressions
In JavaScript, functions are first-class values - they are actually objects. This means that like any other JavaScript type (e.g. numbers or strings) they can be referenced by a variable, passed as an argument to a function, and returned as a value by a function.
Open the functions2.html page in an editor and look at the code.
- 1 - declares two function expressions for formatting a string, and calls them by passing in a string directly
- 2 - declares a function that takes an array and another function as arguments; as the main function loops through the array, the passed in function is called repeatedly
- 2A/B - calls the new function, using an array of strings and each of the two formatting functions from part 1
Preview the file in a browser and check the console output; make sure you understand what's happening. Note the information provided when arrays are written to the console.
Default Function Parameters
In JavaScript, parameters of functions default to undefined. However, in some situations it might be useful to set a different default value. This is where default parameters can help.
Open the functions3.html page in an editor and look at the code. Then preview it in a browser and view the console.
- 1 - When the second parameter is left out, we get a result of
NaN
(Not a Number), because when an undefined value is multiplied by anything, the result is alwaysNaN
- 2 - This function uses a ternary operator, which is a shortcut for if/then/else (
condition ? trueThen : falseElse
), to check to see if a value is undefined. - 3 - Uses default function parameters, which are an ES6 feature
Variable Scope (let & const)
When functions or variables are declared using either let
or var
, they are scoped to the current block they were declared in.
- A Block is delimited by a pair of curly braces
{}
and is used to group statements. - Scope means where your variable is accessible and modifiable in your program.
- Functions and variables declared by
let
andconst
have as their scope the block in which they are defined, as well as in any contained sub-blocks. - If a variable is declared with
let
andconst
outside of a block (at the top-level of the <script> element) it has script scope. Script scope means that the variable is available throughout the current script, and in other <script> tags.
Open the file functions4.html and look at the code. (Note that it begins with the literal statement "use strict", which is an ES5/ES6 command to invoke Strict Mode). Then preview it in a browser. If you're using Chrome, view the "Sources" tab in the developer tools; if you're using Firefox, view the "Debugger" tab. The debugger;
statement will suspend execution of the program and allow you to inspect both the values and the scopes of the variables.
In Chrome, it shows you that you have three scopes here: “Local”, “Script”, and “Global”. In Firefox, it specifies individual functions and blocks, with script being a type of block.
- Ther script scoped variables
A
andB
are available throughout the script block. - The local variables
A
andC
insidefunc1()
, are only available within the function; redeclaringA
here means that changing it in the function does not affect the global value. (It's not great coding practice to have variables with the same name masking each other like this, though; humans tend to get more confused than computers about their values!) - There are also many default globally scoped objects, including alerts, arrays, the
Math
object, and more. All scripts have full access to these and other global variables.
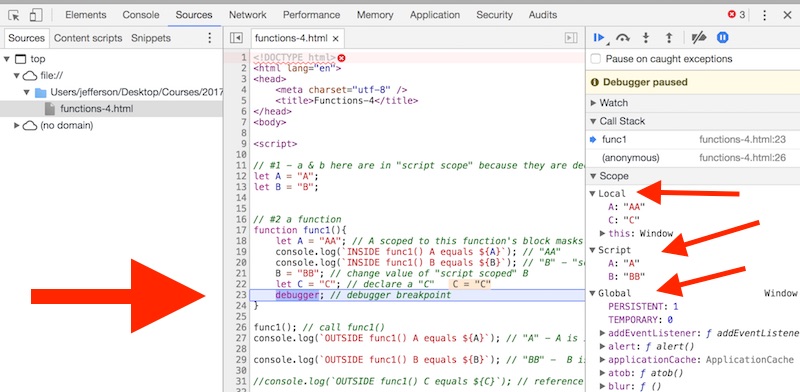
Try this: Remove the debugger;
statement and uncomment the final console.log()
that tries to print out C
. You should get an error message in your console. Do you understand why?
A Few Notes on Variables Defined with var
Variables declared with the var keyword (which preceded the ES6 let and const methods) are scoped to the nearest enclosing function in which they were declared; they are NOT block scoped.
If a variable is declared with the var keyword outside of a function, that variable is now in the global scope rather than the script scope.
You can read about this and other behaviors of the var
keyword--including variable declaration hoisting and global scope--in MDN's var
reference.
Hoisting and the "Temporal Dead Zone"
Function Declaration and Hoisting
Functions that are declared and defined with the function
keyword are available for use within their entire scope, even if code that calls them is placed before they have been declared. Here is an example:
This works because with declared functions, their name and definition (implementation) are both "hoisted" to the top of the scope by the JavaScript runtime, and thus made immediately available to all of the code in that scope.
Function Expressions Declared Using let
But function expressions that are declared with let
will NOT be available.
Other variables declared with let
Other variables declared with let work the same way - the declaration is NOT available:
Variables declared with var
Variables declared with var work differently. The declaration IS hoisted to the top of the function scope, but the definition (assigned value) is NOT:
In this example, x behaves as we expect.
For y, however, here's what will happen:
- The definition of y will be hoisted to the top of the function, but it will be given a value of undefined.
- The ++ operator will be run on the undefined value, which then gives y a value of
NaN
. - The value of 10 is then assigned to y
The Temporal Dead Zone
So what is the "Temporal Dead Zone"? For variables declared with let
or const
, there is a period between entering scope and being declared where they cannot be accessed. This period is known as the temporal dead zone (TDZ). You can read more about it here: JavaScript ES 6: let and the dreaded Temporal Dead Zone.
Deliverable
Part 1
Open the file functions-exercise.html, and review the code. Then make the following changes:
Add at least three of your favorite colors and foods to the arrays. (If you would rather change the theme of the "favorites" to something else--games, music, books etc--feel free.)
Then write two "for" (or "for...in") loops to retrieve the values from the arrays,generating a new list item for each. Refer back to Tuesday's exercises for reminders on how to create a new list item, add content to it, and insert it into the DOM. Instead of inserting it relative to a target item, however, use the appendChild() method to add it to the #colorsList element.
Test your page; you should have two fully populated lists.
Part Two
The problem with the code that you just wrote is that you're duplicating the same basic code for each of the first two lists - this violates a software development best practice known as D.R.Y. - "Don't Repeat Yourself". You need to factor out that duplicated code and put it into a function.
Start with this function declaration:
Replace your two existing for loops with two calls to this function, passing in a reference to the <ol> element you want to populate, and to the relevant array.
When you're done, link the completed file to your landing page as "Week 9 Functions Exercise".