JS Exercise 1: The Console
(Week 9, Tuesday 10/23)
We’re going to start by running some JavaScript in a web page without ever actually displaying anything on the page. Instead, we’ll look at the output of the code in the the JavaScript console window that the browser inspector tools provide.
Hello, World!
As you probably know, Federal law requires that every programming tutorial begin with a “Hello World” application. (Okay, maybe that’s not entirely true. But we’re still going to do it!)
Create a new folder for today’s work, and in create a new HTML document called hello.html. Copy the following code into the body of the document:
Open the file in Chrome or Firefox. It will be blank, since we haven't added any content other than the script tag (which doesn't display on the page). Bring up the console window--in most browsers you have multiple options
- In Chrome or Firefox you can press F12, or you can right click on the page and choose "Inspect" (Chrome) or "Inspect Element" (Firefox), and then select "Console" from the tabs at the top of the Tools panel
- In Chrome you can click on the three vertical dots in the top right corner of the window, and choose More Tools->Developer Tools; in Firefox you can choose the "hamburger" menu, and choose "Web Developer->Web Console"
- There are key combinations available, as well, but they vary across platform and browser, so you'll have to check your specific setup for which keys will work
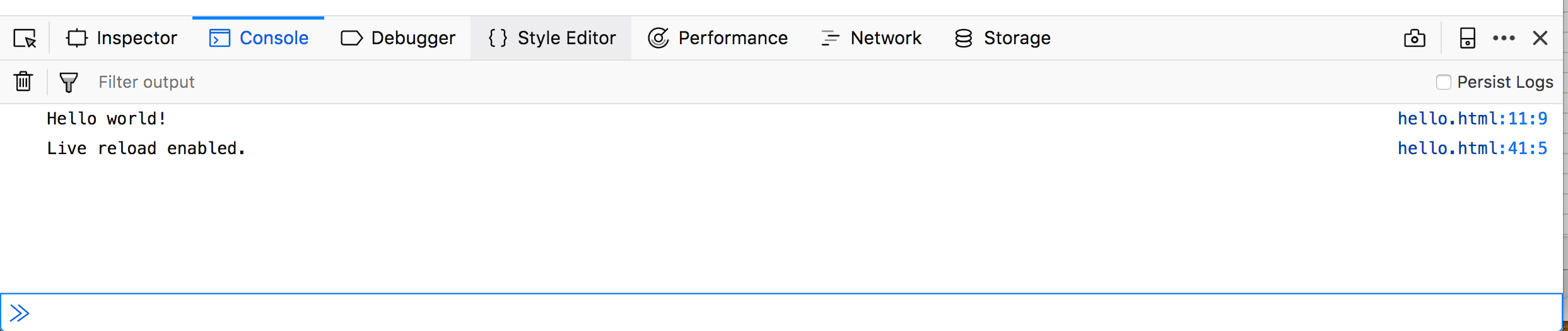
The console.log()
function allows you to output content to the console rather than displaying it on the page. You should see the output of your script in the console window. (If you're using a live preview function from your editor, you may also see other Javascript output that's been added.) This can be extremely helpful when debugging your code!
The console also contains an interactive interpreter where you can run JavaScript commands. At the prompt, type Date()
to create and see a new date from the Date
object, and Math.random()
to get a random number from the Math
object.
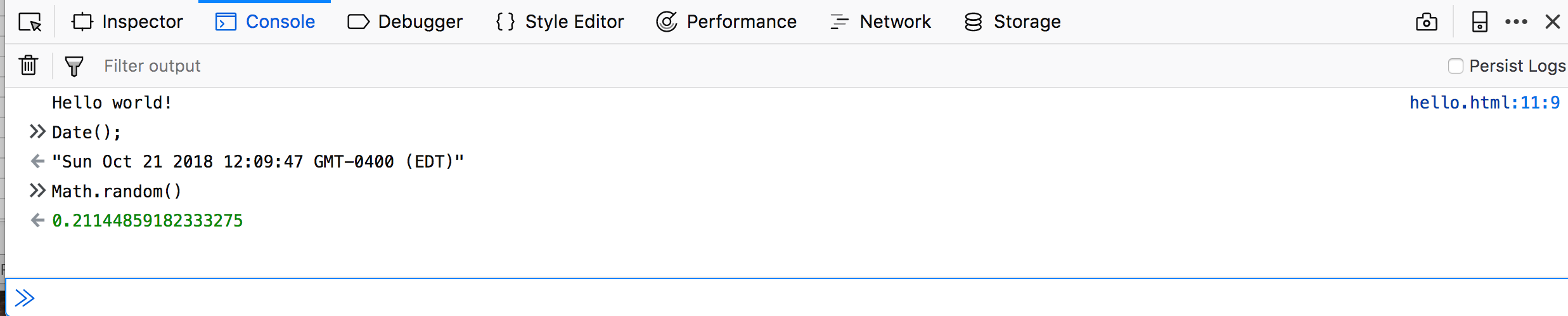
Note: We're not making changes to the HTML file when we enter commands into the console; we’re just testing out what our code would do.
Declaring Variables and Constants
In pre-ES6 versions of JavaScript, all variables were defined with the var
keyword, and you'll still see that in a lot of code libraries and examples online. In ES6, we can use the const
keyword to declare constant values (that do not change), and the let
keyword to declare variables.
Many developers (and several IGM professors) recommend that you NOT use the pre-ES6 approach of var
to declare variables, as the variables that var
declares are scoped to functions, rather than the block scoping of let
and const
, which introduces odd behavior. However, if your code needs to work on older browsers, you may still need to use var. Read more here: Why You Shouldn't Use var Anymore
JavaScript is a loosely typed (aka dynamic) language. That means you don’t have to declare the type of a variable ahead of time. The type will be determined automatically by the engine while the program is being run. That also means that the same variable can contain data of different types
After the console.log command in your <script> block, add the following lines:
View the file in a browser. Again, you’ll see nothing in the browser window, but in the console you should see this:

Some things to note about that code: - When strings are added to numbers, we get back a concatenated string. - ES6 also allows String Templating, which is more powerful than simple string concatenation. Note the backtick ( ` ) symbol is used to denote the string, and ${}
encloses the variable names.
Console Error Messages
The console will display error messages that will help you debug your code. Let’s produce an error by attempting to change the value of the answer
constant above. Add this line to the end of your script block:
When you reload the page in the browser, you should see an error in the console:

(The screenshot is from Firefox; in Chrome, the error will read "hello-2.html:19 Uncaught TypeError: Assignment to constant variable.")
That’s because we defined answer
as a constant rather than a variable. Fix this error by changing the declaration for answer
to a variable rather than a constant.
Data Types and Objects
The five common built-in “primitive” data types in JavaScript are: Number, String, Boolean, Undefined (a value has never been defined) and Null (the intentional absence of a value). ES6 has also added the Symbol type.
Replace the contents of your script block with the following code:
View the file in the browser again. You should see the string “Joe” output to the console.
JavaScript also contains a number of built-in objects that we can use. There is Object
, which is a starting point for our own customized objects, as well as Array
, Date
, Math
, and others. The “primitive” types above also can be treated like objects and have properties and methods that can be called on them.
- Arrays - A list of values
- Objects - A list of name/value pairs
- Date - Represents a single moment in time
- Math - A built-in object with properties and methods for mathematical operations
Objects have methods and properties that you can access with your code. For instance:
- obj.toString()- Returns a string representing the object.
- obj.toFixed() - Formats a number using fixed-point notation.
- str.length - Returns the length of a string.
In your hello.html document, replace the contents of the script block with this:
preview the file in Chrome and view the console. You should see nine values output, and they should match the values in the code comments. Make sure you understand how each of those values is being generated!
Submitting Your Work
Link your hello.html file from your landing page as JS Console Exercise, and publish it to your GitHub site. This should be done before the end of class.